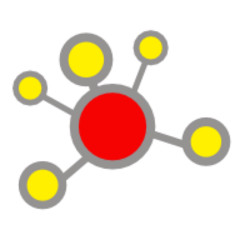
Generate random graphs according to the random dot product graph model
Source:R/games.R
sample_dot_product.Rd
In this model, each vertex is represented by a latent position vector. Probability of an edge between two vertices are given by the dot product of their latent position vectors.
Details
The dot product of the latent position vectors should be in the [0,1] interval, otherwise a warning is given. For negative dot products, no edges are added; dot products that are larger than one always add an edge.
References
Christine Leigh Myers Nickel: Random dot product graphs, a model for social networks. Dissertation, Johns Hopkins University, Maryland, USA, 2006.
See also
sample_dirichlet()
, sample_sphere_surface()
and sample_sphere_volume()
for sampling position vectors.
Random graph models (games)
erdos.renyi.game()
,
sample_()
,
sample_bipartite()
,
sample_chung_lu()
,
sample_correlated_gnp()
,
sample_correlated_gnp_pair()
,
sample_degseq()
,
sample_fitness()
,
sample_fitness_pl()
,
sample_forestfire()
,
sample_gnm()
,
sample_gnp()
,
sample_grg()
,
sample_growing()
,
sample_hierarchical_sbm()
,
sample_islands()
,
sample_k_regular()
,
sample_last_cit()
,
sample_pa()
,
sample_pa_age()
,
sample_pref()
,
sample_sbm()
,
sample_smallworld()
,
sample_traits_callaway()
,
sample_tree()
Author
Gabor Csardi csardi.gabor@gmail.com
Examples
## A randomly generated graph
lpvs <- matrix(rnorm(200), 20, 10)
lpvs <- apply(lpvs, 2, function(x) {
return(abs(x) / sqrt(sum(x^2)))
})
g <- sample_dot_product(lpvs)
g
#> IGRAPH 158361a U--- 10 30 --
#> + edges from 158361a:
#> [1] 1-- 2 1-- 3 1-- 5 1-- 6 1-- 7 1-- 8 1--10 2-- 4 2-- 6 2-- 7 2--10 3-- 4
#> [13] 3-- 7 3-- 8 3-- 9 3--10 4-- 5 4-- 6 4-- 7 4-- 8 4-- 9 4--10 5-- 6 5-- 9
#> [25] 6-- 7 6-- 8 6--10 7--10 8-- 9 9--10
## Sample latent vectors from the surface of the unit sphere
lpvs2 <- sample_sphere_surface(dim = 5, n = 20)
g2 <- sample_dot_product(lpvs2)
g2
#> IGRAPH e952bd9 U--- 20 146 --
#> + edges from e952bd9:
#> [1] 1-- 2 1-- 5 1-- 6 1-- 7 1-- 8 1-- 9 1--10 1--11 1--12 1--13 1--14 1--15
#> [13] 1--16 1--17 1--18 1--20 2-- 3 2-- 5 2-- 6 2-- 7 2-- 8 2-- 9 2--12 2--13
#> [25] 2--14 2--16 2--17 2--18 2--19 2--20 3-- 4 3-- 5 3-- 6 3-- 7 3-- 9 3--11
#> [37] 3--12 3--13 3--14 3--16 3--17 3--18 3--19 3--20 4-- 5 4-- 7 4--10 4--11
#> [49] 4--12 4--13 4--14 4--15 4--17 4--19 4--20 5-- 6 5-- 7 5-- 8 5-- 9 5--10
#> [61] 5--12 5--13 5--16 5--17 5--18 5--19 6-- 7 6-- 8 6--10 6--11 6--12 6--13
#> [73] 6--14 6--15 6--16 6--17 6--19 7-- 8 7-- 9 7--10 7--11 7--12 7--13 7--14
#> [85] 7--16 7--18 7--19 7--20 8-- 9 8--10 8--12 8--13 8--14 8--15 8--16 8--17
#> [97] 8--20 9--11 9--12 9--13 9--14 9--15 9--16 9--17 9--18 9--19
#> + ... omitted several edges